Connect the "SCL" of the model to D1 pin of the esp8266 and the "SDA" to D2, some people put a pull-up resistor but it's work anyway so it up to you.
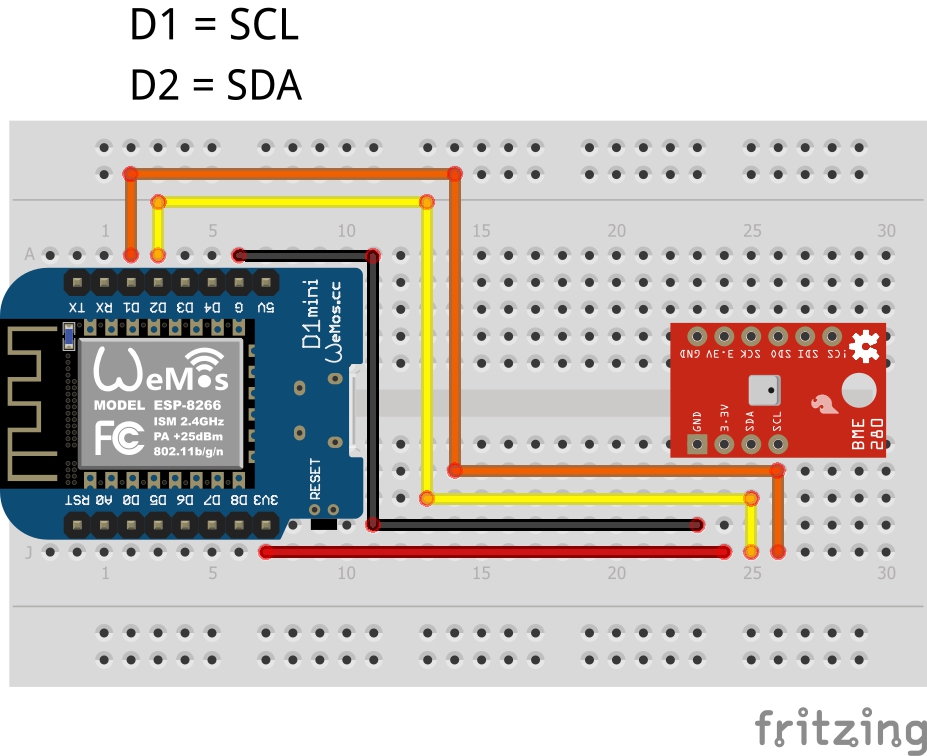
after that upload the sketch to the esp8266 and open the serial monitor to see the ip address of the esp.
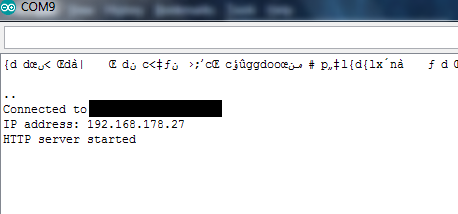
as you see my esp8266 transmit data on ip number 192.168.178.27, be aware maybe you have a different ip address so always open the serial monitor to make sure your data transmission web page.
after that, the web page looks like this and it will be updated automatically every 10 seconds with new data.
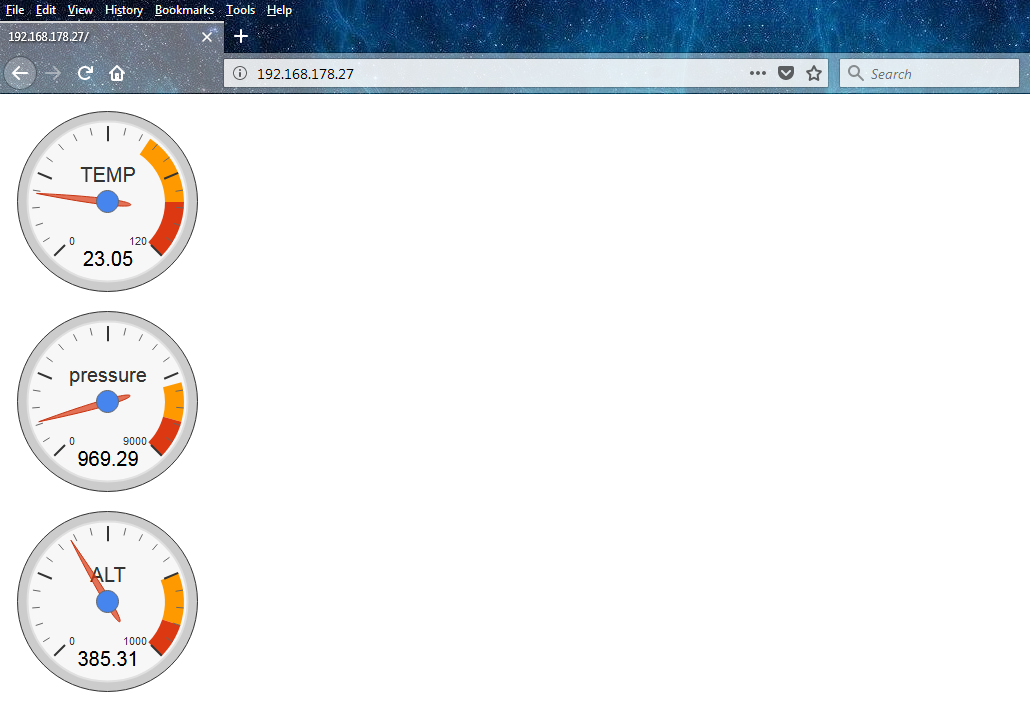
Arduino Code
#include <ESP8266WiFi.h> #include <ESP8266WebServer.h> #include <stdint.h> #include "SparkFunBME280.h" #include "Wire.h" //Global sensor object BME280 mySensor; const char* ssid = "your wifi SSID"; const char* password = "your wifi password"; ESP8266WebServer server (80); String INDEX_HTML=""; const int led = D7; void setup () { Serial.begin ( 115200 ); // init for the sensor mySensor.settings.commInterface = I2C_MODE; mySensor.settings.I2CAddress = 0x76; mySensor.settings.runMode = 3; mySensor.settings.tStandby = 0; mySensor.settings.filter = 0; mySensor.settings.tempOverSample = 1; mySensor.settings.pressOverSample = 1; mySensor.settings.humidOverSample = 1; delay(200); Serial.println(mySensor.begin(), HEX); // Webserver pinMode ( led, OUTPUT ); digitalWrite ( led, 0 ); WiFi.begin ( ssid, password ); Serial.println ( "" ); // Wait for connection while ( WiFi.status() != WL_CONNECTED ) { delay ( 500 ); Serial.print ( "." ); } Serial.println ( "" ); Serial.print ( "Connected to " ); Serial.println ( ssid ); Serial.print ( "IP address: " ); Serial.println ( WiFi.localIP() ); // what to do with requests server.on ( "/", handleRoot ); server.onNotFound ( handleNotFound ); server.begin(); Serial.println ( "HTTP server started" ); } void loop () { // waiting fo a client server.handleClient(); } void handleRoot() { INDEX_HTML=""; Serial.println("Client connected"); digitalWrite ( led, 1 ); INDEX_HTML= "<!DOCTYPE HTML>" "<head>" "<meta http-equiv=\"refresh\" content=\"10\">" "<script type=\"text/javascript\" src=\"https://www.gstatic.com/charts/loader.js\"></script>" "<script type=\"text/javascript\">" "google.charts.load('current', {'packages':['gauge']});" "google.charts.setOnLoadCallback(drawGauge);" "var gaugeOptions = {min: 0, max: 120, yellowFrom: 75, yellowTo: 100," "redFrom: 100, redTo: 120, minorTicks: 5};" "var gaugeOptionsp = {min: 0, max: 9000, yellowFrom: 7000, yellowTo: 8000," "redFrom: 8000, redTo: 9000, minorTicks: 5};" "var gaugeOptionsa = {min: 0, max: 1000, yellowFrom: 750, yellowTo: 900," "redFrom: 900, redTo: 1000, minorTicks: 5};" "var gauge;" "function drawGauge() {" "gaugeData = new google.visualization.DataTable();" "gaugeData1 = new google.visualization.DataTable();" "gaugeData2 = new google.visualization.DataTable();" "gaugeData.addColumn('number', 'TEMP');" "gaugeData1.addColumn('number', 'pressure');" "gaugeData2.addColumn('number', 'ALT');" "gaugeData.addRows(3);" "gaugeData1.addRows(3);" "gaugeData2.addRows(3);" "gaugeData.setCell(0, 0, 0);" "gaugeData1.setCell(1, 0, 0);" "gaugeData2.setCell(2, 0, 0);" "gauge = new google.visualization.Gauge(document.getElementById('gauge_div'));" "gaugeData.setValue(0, 0,"; INDEX_HTML+=String(mySensor.readTempC(), 2); INDEX_HTML+=");" "gauge.draw(gaugeData, gaugeOptions);" "gauge = new google.visualization.Gauge(document.getElementById('gauge_div1'));" "gaugeData1.setValue(1, 0,"; INDEX_HTML+=String(mySensor.readFloatPressure()/100); INDEX_HTML+=");" "gauge.draw(gaugeData1, gaugeOptionsp);" "gauge = new google.visualization.Gauge(document.getElementById('gauge_div2'));" "gaugeData2.setValue(2, 0,"; INDEX_HTML+=String(mySensor.readFloatAltitudeMeters(), 2); INDEX_HTML+=");" "gauge.draw(gaugeData2, gaugeOptionsa);" "}" "</script>" "</head>" "<body>" "<div id=\"gauge_div\" style=\"width:580px; height: 200px;\"></div>" "<div id=\"gauge_div1\" style=\"width:580px; height: 200px;\"></div>" "<div id=\"gauge_div2\" style=\"width:580px; height: 200px;\"></div>" "</body>" "</html>"; server.send(200, "text/html", INDEX_HTML); digitalWrite ( led, 0 ); } void handleNotFound() { digitalWrite ( led, 1 ); String message = "File Not Found\n\n"; message += "URI: "; message += server.uri(); message += "\nMethod: "; message += ( server.method() == HTTP_GET ) ? "GET" : "POST"; message += "\nArguments: "; message += server.args(); message += "\n"; for ( uint8_t i = 0; i < server.args(); i++ ) { message += " " + server.argName ( i ) + ": " + server.arg ( i ) + "\n"; } server.send ( 404, "text/plain", message ); digitalWrite ( led, 0 ); }