First, you need an app called "Serial Bluetooth Terminal" to make a link between your esp32 and phone via Bluetooth to choose the wifi network and to send the password of your network.
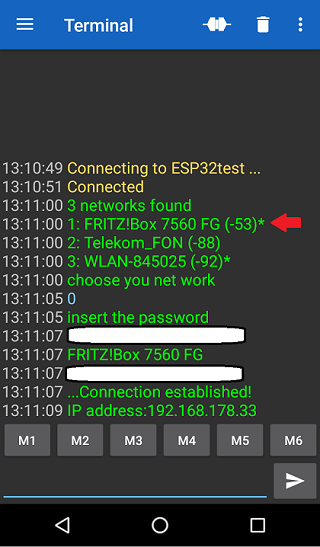
as you see in the photo I managed to contact my router by choosing the number of my network which is 0 because the counting starts from 0 not from 1 so be aware of it.
Second, go to your browser and write the IP address as shown in the App and then the web server appears and after that, you can control the Leds by the browser.
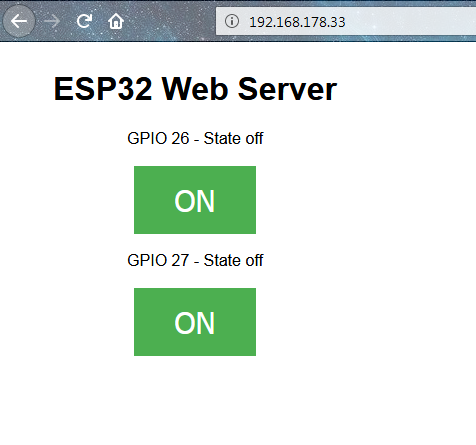
Note: this program it's can't save the password but you can add a library
Arduino ESP32 code
#include <WiFi.h> #include "BluetoothSerial.h" String val1; String val2; String w[4]; #if !defined(CONFIG_BT_ENABLED) || !defined(CONFIG_BLUEDROID_ENABLED) #error Bluetooth is not enabled! Please run `make menuconfig` to and enable it #endif BluetoothSerial SerialBT; WiFiServer server(80); String header; String output26State = "off"; String output27State = "off"; const int output26 = 26; const int output27 = 27; void wifi() { SerialBT.println("choose you network"); while(SerialBT.available()==0); { val1=SerialBT.readStringUntil('\r'); delay(500); SerialBT.flush(); } SerialBT.println("Enter the password"); while(SerialBT.available()==0); { val2=SerialBT.readStringUntil('\r'); delay(500); SerialBT.flush(); } val1=w[val1.toInt()]; SerialBT.println(val1.c_str()); SerialBT.println(val2.c_str()); WiFi.begin(val1.c_str(),val2.c_str()); while (WiFi.status() != WL_CONNECTED) { SerialBT.print("."); delay(500); } } void BL() { // Set WiFi to station mode and disconnect from an AP if it was previously connected delay(100); // WiFi.scanNetworks will return the number of networks found int n = WiFi.scanNetworks(); if (n == 0) { SerialBT.println("no networks found"); } else { SerialBT.print(n); SerialBT.println(" networks found"); for (int i = 0; i < n; ++i) { // Print SSID and RSSI for each network found SerialBT.print(i + 1); SerialBT.print(": "); SerialBT.print(WiFi.SSID(i)); w[i]=WiFi.SSID(i); SerialBT.print(" ("); SerialBT.print(WiFi.RSSI(i)); SerialBT.print(")"); SerialBT.println((WiFi.encryptionType(i) == WIFI_AUTH_OPEN)?" ":"*"); delay(10); } } } void setup() { delay(2000); SerialBT.begin("ESP32test"); //Bluetooth device name SerialBT.println("The device started, now you can pair it with bluetooth!"); delay(7000); Serial.begin(115200); BL(); wifi(); SerialBT.println("Connection established!"); SerialBT.print("IP address:"); SerialBT.println(WiFi.localIP()); pinMode(output26, OUTPUT); pinMode(output27, OUTPUT); // Set outputs to LOW digitalWrite(output26, LOW); digitalWrite(output27, LOW); server.begin(); } void loop() { WiFiClient client = server.available(); // Listen for incoming clients if (client) { // If a new client connects, Serial.println("New Client."); // print a message out in the serial port String currentLine = ""; // make a String to hold incoming data from the client while (client.connected()) { // loop while the client's connected if (client.available()) { // if there's bytes to read from the client, char c = client.read(); // read a byte, then Serial.write(c); // print it out the serial monitor header += c; if (c == '\n') { // if the byte is a newline character // if the current line is blank, you got two newline characters in a row. // that's the end of the client HTTP request, so send a response: if (currentLine.length() == 0) { // HTTP headers always start with a response code (e.g. HTTP/1.1 200 OK) // and a content-type so the client knows what's coming, then a blank line: client.println("HTTP/1.1 200 OK"); client.println("Content-type:text/html"); client.println("Connection: close"); client.println(); // turns the GPIOs on and off if (header.indexOf("GET /26/on") >= 0) { Serial.println("GPIO 26 on"); output26State = "on"; digitalWrite(output26, HIGH); } else if (header.indexOf("GET /26/off") >= 0) { Serial.println("GPIO 26 off"); output26State = "off"; digitalWrite(output26, LOW); } else if (header.indexOf("GET /27/on") >= 0) { Serial.println("GPIO 27 on"); output27State = "on"; digitalWrite(output27, HIGH); } else if (header.indexOf("GET /27/off") >= 0) { Serial.println("GPIO 27 off"); output27State = "off"; digitalWrite(output27, LOW); } // Display the HTML web page client.println("<!DOCTYPE html><html>"); client.println("<head><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">"); client.println("<link rel=\"icon\" href=\"data:,\">"); // CSS to style the on/off buttons // Feel free to change the background-color and font-size attributes to fit your preferences client.println("<style>html { font-family: Helvetica; display: inline-block; margin: 0px auto; text-align: center;}"); client.println(".button { background-color: #4CAF50; border: none; color: white; padding: 16px 40px;"); client.println("text-decoration: none; font-size: 30px; margin: 2px; cursor: pointer;}"); client.println(".button2 {background-color: #555555;}</style></head>"); // Web Page Heading client.println("<body><h1>ESP32 Web Server</h1>"); // Display current state, and ON/OFF buttons for GPIO 26 client.println("<p>GPIO 26 - State " + output26State + "</p>"); // If the output26State is off, it displays the ON button if (output26State=="off") { client.println("<p><a href=\"/26/on\"><button class=\"button\">ON</button></a></p>"); } else { client.println("<p><a href=\"/26/off\"><button class=\"button button2\">OFF</button></a></p>"); } // Display current state, and ON/OFF buttons for GPIO 27 client.println("<p>GPIO 27 - State " + output27State + "</p>"); // If the output27State is off, it displays the ON button if (output27State=="off") { client.println("<p><a href=\"/27/on\"><button class=\"button\">ON</button></a></p>"); } else { client.println("<p><a href=\"/27/off\"><button class=\"button button2\">OFF</button></a></p>"); } client.println("</body></html>"); // The HTTP response ends with another blank line client.println(); // Break out of the while loop break; } else { // if you got a newline, then clear currentLine currentLine = ""; } } else if (c != '\r') { // if you got anything else but a carriage return character, currentLine += c; // add it to the end of the currentLine } } } // Clear the header variable header = ""; // Close the connection client.stop(); Serial.println("Client disconnected."); Serial.println(""); } }