the gpio output voltage is 3.3v and the same in input signal also the maximum output current 30ma so it can drive a led diode but it will be batter if you use a transistor to protect the pin , as well any 5v signal it will be damaged the board so be aware of that.
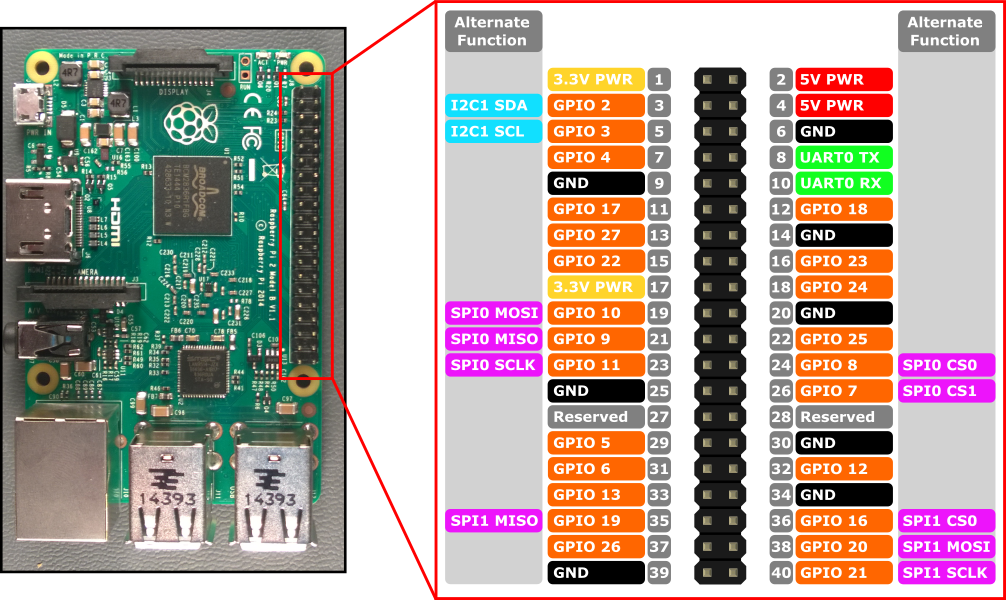
so as you see the gpio have UART port for serial communication , I2C port, SPI and the rest of these it's a input or output pin that depending how you want to program it.
lessons
lesson 1:
make the pins number 16,18,22 an output and toggle it with delay 3 seconds.import time import RPi.GPIO as GPIO GPIO.setmode(GPIO.BOARD) GPIO.setup(22,GPIO.OUT) GPIO.setup(16,GPIO.OUT) GPIO.setup(18,GPIO.OUT) GPIO.output(22,GPIO.HIGH) GPIO.output(18,GPIO.HIGH) GPIO.output(16,GPIO.HIGH) time.sleep(3) GPIO.output(16,GPIO.LOW) GPIO.output(18,GPIO.LOW) GPIO.output(22,GPIO.LOW) GPIO.cleanup() print "DONE"
lesson 2:
make the gpio 16,18,22 an output but this time you can control its status either "on" or "off".import RPi.GPIO as GPIO import time pin_1=16 pin_2=18 pin_3=22 GPIO.setmode(GPIO.BOARD) GPIO.setup(pin_1,GPIO.OUT) GPIO.setup(pin_2,GPIO.OUT) GPIO.setup(pin_3,GPIO.OUT) GPIO.output(pin_1,GPIO.LOW) GPIO.output(pin_2,GPIO.LOW) GPIO.output(pin_3,GPIO.LOW) while 1: re=raw_input("Enter the pin you want ON :- ") if re == "1": GPIO.output(pin_1,GPIO.HIGH) if re == "2": GPIO.output(pin_2,GPIO.HIGH) if re == "3": GPIO.output(pin_3,GPIO.HIGH) if re== "off all": GPIO.output(pin_1,GPIO.LOW) GPIO.output(pin_2,GPIO.LOW) GPIO.output(pin_3,GPIO.LOW) if re == "out": GPIO.cleanup() break
lesson 3:
how can we check the status of the pin and do something if the reading high or low.import RPi.GPIO as GPIO import time pin_1=16 pin_2=18 pin_3=22 GPIO.setmode(GPIO.BOARD) GPIO.setup(pin_1,GPIO.OUT) GPIO.setup(pin_2,GPIO.OUT) GPIO.setup(pin_3,GPIO.OUT) GPIO.output(pin_1,GPIO.LOW) GPIO.output(pin_2,GPIO.LOW) GPIO.output(pin_3,GPIO.LOW) print ("Enter 1,2,3 for leds and out to exit") while 1: re=raw_input("Enter the pin you want ON :- ") if re == "1": if GPIO.input(pin_1): GPIO.output(pin_1,GPIO.LOW) else: GPIO.output(pin_1,GPIO.HIGH) if re == "2": if GPIO.input(pin_2): GPIO.output(pin_2,GPIO.LOW) else: GPIO.output(pin_2,GPIO.HIGH) if re == "3": if GPIO.input(pin_3): GPIO.output(pin_3,GPIO.LOW) else: GPIO.output(pin_3,GPIO.HIGH) if re == "out": GPIO.cleanup() break
lesson 4:
generate PWM signal from pin 22 to control for example speed of a motor or the angle of the servo motor and more.import RPi.GPIO as GPIO import time pwm_pin=22 GPIO.setmode(GPIO.BOARD) GPIO.setup(pwm_pin,GPIO.OUT) pwm=GPIO.PWM(pwm_pin,50) pwm.start(0) print ("Enter number for pwm from 0-100") print ("Enter out for exit") while 1: p=raw_input("Enter the pwm value:- ") if p =="out": pwm.stop() GPIO.cleanup() print "DONE" break else: dc= float(p) pwm.ChangeDutyCycle(dc)
lesson 5:
activated the pull-up resistors and change the status of the pin every time pressing the switch that connects to pin 26.#this is toggle program import RPi.GPIO as GPIO GPIO.setmode(GPIO.BOARD) GPIO.setup(26,GPIO.IN,pull_up_down=GPIO.PUD_UP) GPIO.setup(22,GPIO.OUT) GPIO.output(22,GPIO.LOW) try: while 1: if (GPIO.input(26)==0) and (GPIO.input(22)==1): GPIO.output(22,GPIO.LOW) GPIO.wait_for_edge(26,GPIO.RISING) if (GPIO.input(26)==0) and (GPIO.input(22)==0): GPIO.output(22,GPIO.HIGH) GPIO.wait_for_edge(26,GPIO.RISING) except KeyboardInterrupt: GPIO.cleanup() print "DONE"
lesson 6:
this code will print a message when you press the button that connects to pin 26 and waiting in an empty loop until button released.import RPi.GPIO as GPIO GPIO.setmode(GPIO.BOARD) GPIO.setup(26,GPIO.IN,pull_up_down=GPIO.PUD_UP) coun=0 while 1: GPIO.wait_for_edge(26,GPIO.FALLING) print "key has been press" GPIO.wait_for_edge(26,GPIO.RISING) print "key has been released" coun+=1 if coun==10: GPIO.cleanup() break